Trajectory Generator#
To ensure repeatability of measurements, trajectories are a fundamental tool. The present software module can generate setvalues based on defined grid points and simplify the operational management of the system. Various interpolation methodologies, generation options and start/stop behaviors are available to customize operation control.
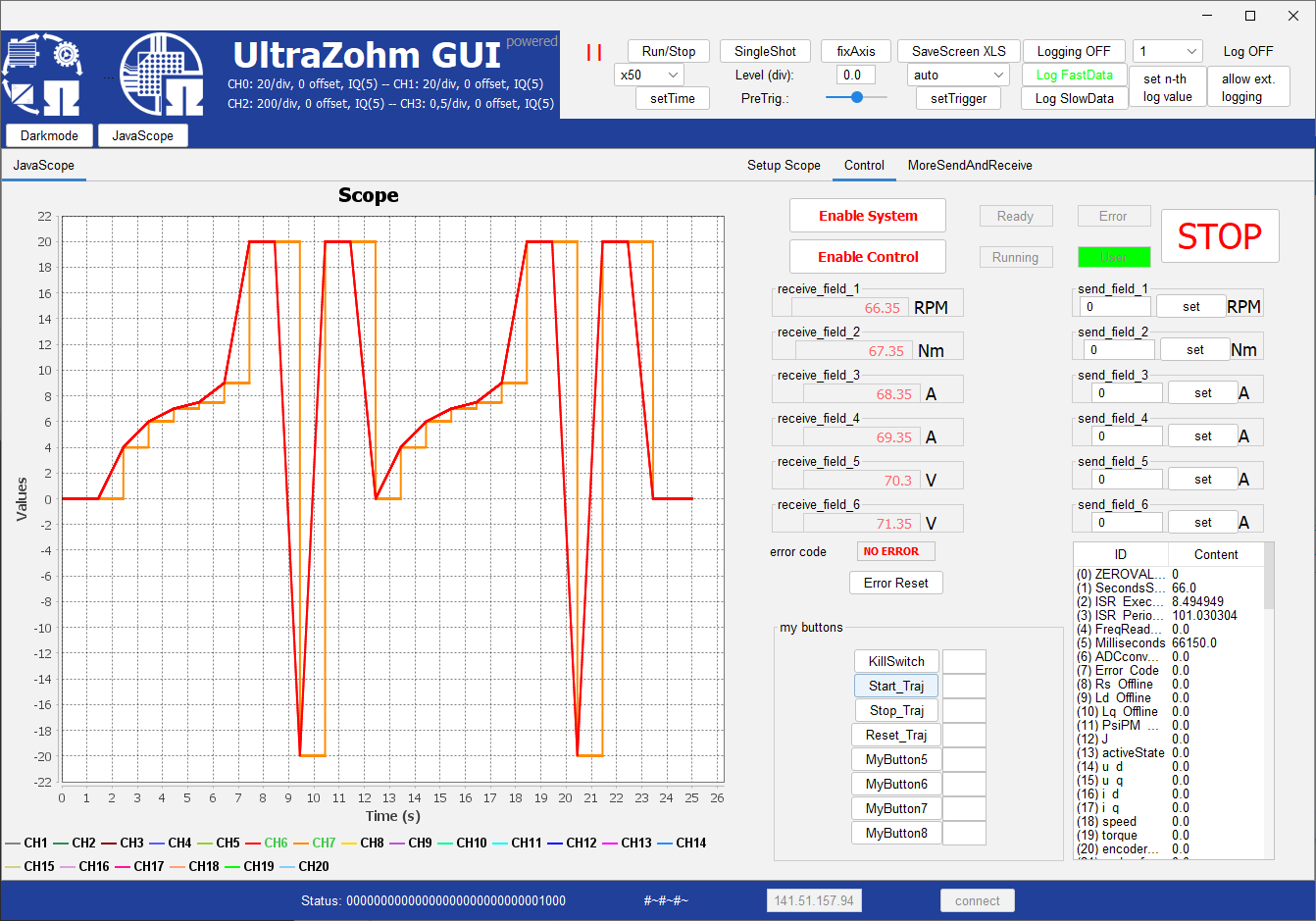
Fig. 311 Example-Trajectory
Example-Trajectories on the JavaScope - Linear interpolation red . Zero-Order-Hold orange#
Setup#
Defines#
Inside the uz_Trajectory.h
is a define , which limits the maximum number of samples.
This must first be set to the desired number of data points before the module can be configured.
-
MAX_TRAJECTORY_SAMPLES#
Maximum number of samples that can be stored. Should be as small as possible to save resources.
Struct#
Struct to store the desired configuration.
-
typedef struct uz_Trajectory_t uz_Trajectory_t#
Object definition for uz_Trajectory_t.
Configuration#
The trajectory generator is able to use different interpolation algorithms between the single data points. Currently, only no interpolation (zero-order hold) or linear interpolation is implemented.
-
enum uz_Trajectory_interpolation_selection#
enum for readable configuration of the interpolation type
Values:
-
enumerator Zero_Order_Hold#
-
enumerator Linear#
-
enumerator Zero_Order_Hold#
Select the unit of the time base. The values for time are durations, so only values greater than zero will result in correct behavior.
-
enum uz_Trajectory_XTicks_selection#
enum for readable configuration of the X-Axis-Style
Values:
-
enumerator ISR_Ticks#
-
enumerator MicroSeconds#
-
enumerator MilliSeconds#
-
enumerator Seconds#
-
enumerator ISR_Ticks#
Switch to force the generator to repeat the sequence infinitely or a specified number of times.
-
enum uz_Trajectory_Repeat_selection#
enum for readable configuration of the repeat behavior
Values:
-
enumerator Repeat_Times#
-
enumerator Repeat_Inf#
-
enumerator Repeat_Times#
Switch for setting the output behavior. It is possible to hold the last value or a defined zero point when the trajectory has stopped or passed through.
-
enum uz_Trajectory_Stop_Output_selection#
enum for readable configuration of the output behavior
Values:
-
enumerator ForceToZero#
-
enumerator HoldLast#
-
enumerator ForceToZero#
Config struct to create the trajectory generator
-
struct uz_Trajectory_config#
configuration struct for a trajectory generator.
Public Members
-
enum uz_Trajectory_interpolation_selection selection_interpolation#
Interpolation type selection
-
enum uz_Trajectory_XTicks_selection selection_XAxis#
X-Axis-Style selection
-
enum uz_Trajectory_Stop_Output_selection StopStyle#
Defines the behavior when reaching the end or trajectory is stopped
-
enum uz_Trajectory_Repeat_selection RepeatStyle#
Defines whether the playback process is to be repeated x times or infinitely
-
uint32_t Number_Sample_Points#
Number of samples of the signal to be used. Can be used to display only the first X values.
If this feature isn’t needed, set his value to MAX_TRAJECTORY_SAMPLES
-
float Sample_Amplitude_Y[MAX_TRAJECTORY_SAMPLES]#
Y-Axis-Amplitude of the signal
-
float Sample_Duration_X[MAX_TRAJECTORY_SAMPLES]#
X-Axis-Duration of each Sample of the signal
-
uint32_t Repeats#
Number of repeats
-
float Stepwidth_ISR#
Time of the Stepwidth in with the Trajectory is called
-
enum uz_Trajectory_interpolation_selection selection_interpolation#
Example#
1#include "uz/uz_Trajectory/uz_Trajectory.h"
2int main(void) {
3 struct uz_Trajectory_config Trace1_Config = {
4 .selection_interpolation = Linear,
5 .selection_XAxis = MilliSeconds,
6 .StopStyle = HoldLast,
7 .RepeatStyle = Repeat_Times,
8 .Number_Sample_Points = 11.0f,
9 .Sample_Amplitude_Y = {0.0f, 4.0f, 6.0f, 7.0f, 7.5f, 9.0f, 20.0f, 20.0f, -20.0f, 20.0f, 20.0f},
10 .Sample_Duration_X = {1000.0f, 1000.0f, 1000.0f, 1000.0f, 1000.0f, 1000.0f, 1000.0f, 1000.0f, 1000.0f, 1000.0f , 1000.0f},
11 .Repeats = 2,
12 .Stepwidth_ISR = (1.0f / UZ_PWM_FREQUENCY) * (Interrupt_ISR_freq_factor)
13 };
14}
Init function#
-
uz_Trajectory_t *uz_Trajectory_init(struct uz_Trajectory_config config)#
Initialization of the Trajectory object.
- Parameters:
config – uz_Trajectory_config configuration struct
- Returns:
Pointer to uz_Trajectory_t object
Example#
config
according to configuration section#1int main(void) {
2 uz_Trajectory_t* Traj_instance = uz_Trajectory_init(Traj_config);
3}
Description#
Allocates the memory for the trajectory generator instance. Furthermore the input values of the configuration struct are asserted.
Functions#
Predefined functions are available for easier operation.
The Start, Stop and Reset functions can be called directly from the ipc_ARM.c
file and should be used to control the trajectory generator.
Start#
-
void uz_Trajectory_Start(uz_Trajectory_t *self)#
Starts the trajectory.
- Parameters:
self – pointer to uz_Trajectory_t object
Example#
1#include "uz/uz_Trajectory/uz_Trajectory.h"
2void ipc_Control_func(uint32_t msgId, float value, DS_Data *data){
3 ...
4 case (My_Button_2):
5 ultrazohm_state_machine_set_userLED(true);
6 uz_Trajectory_Start(Global_Data.objects.TraceGen_1);
7 break;
8 ...
9}
Stop#
-
void uz_Trajectory_Stop(uz_Trajectory_t *self)#
Stops the trajectory.
- Parameters:
self – pointer to uz_Trajectory_t object
Example#
1#include "uz/uz_Trajectory/uz_Trajectory.h"
2void ipc_Control_func(uint32_t msgId, float value, DS_Data *data){
3 ...
4 case (My_Button_3):
5 ultrazohm_state_machine_set_userLED(false);
6 uz_Trajectory_Stop(Global_Data.objects.TraceGen_1);
7 break;
8 ...
9}
Reset#
-
void uz_Trajectory_Reset(uz_Trajectory_t *self)#
Resets the trajectory.
- Parameters:
self – pointer to uz_Trajectory_t object
Example#
1#include "uz/uz_Trajectory/uz_Trajectory.h"
2void ipc_Control_func(uint32_t msgId, float value, DS_Data *data){
3 ...
4 case (My_Button_4):
5 ultrazohm_state_machine_set_userLED(false);
6 uz_Trajectory_Reset(Global_Data.objects.TraceGen_1);
7 break;
8 ...
9}
The Step-function should be called from the ISR inside the file isr.c
.
Step#
-
float uz_Trajectory_Step(uz_Trajectory_t *self)#
Outputs the calculated trajectory.
- Parameters:
self – pointer to uz_Trajectory_t object
- Returns:
float Returns current trajectory step
Example#
1#include "uz/uz_Trajectory/uz_Trajectory.h"
2void ISR_Control(void *data){
3 ...
4 // Generate Trajectory
5 Global_Data.av.Traj_1 = uz_Trajectory_Step(Global_Data.objects.TraceGen_1);
6 ...
7}
Usecase#
To create a trajectory with the generator module and use it in operation, the following steps must be followed:
Define the needed trajectory and determine the grid points.
Define the Samples inside the uz_trajectory.h.
Write the configuration and create the trajectory generator object.
If the complete trajectory is to be played back, the value
config.Number_Sample_Points
can be set to the defineMAX_TRAJECTORY_SAMPLES
. Otherwise, the first X grid points can be played with the valueconfig.Number_Sample_Points
.
Add the functions uz_Trajectory_Start, uz_Trajectory_Stop and uz_Trajectory_Reset to the IPC-Handler.
It is recommended to define some buttons of the JavaScope to control the trajectory generator and to label the buttons according to the programmed functionality.
Add the function uz_Trajectory_Step to the ISR and create a variable for the trajectory inside the Global_Data.
Add the Output of the trajectory generator to the Global_Data and the JavaScope.
Compile the Code and Start the UltraZohm.
After you set up the trajectory generator, you can control the behavior using the JavaScope buttons and play your own trajectories. To do this, first call the start function. The generator will start playing the trajectory until the termination condition is reached or continue doing so indefinitely. The trajectory can be stopped with the Stop function. If the trajectory is to be continued, the start function must be called up again. When the end of the trajectory is reached in repeat mode, the generator locks automatically to prevent an accidental second run. If a second run is desired, the trajectory must first be unlocked with the Reset function.
Designed by#
Robert Zipprich (University of Kassel Kassel / EMA) in 10/2023