Space vector Modulation 6ph#
General#
Note
This module implements a space vector modulation for asymmetrical six-phase machine with isolated neutral points (2N). The implementation is adaptable to 1N and described in the references.
SVM for multi-phase works similar to Space vector Modulation. However, in a multi-phase network, more switching combinations are available (for six-phase \(2^6=64\)). Furthermore, switching combination has simultaniously one space vector (SV) in the \(\alpha\beta\)- and one in the \(XY\)-subspace. During the calculations, both have to be taken account of and it has to be noted, that a combination with a large SV (high absolute value) in \(\alpha\beta\) means usually a small SV in \(XY\). Since an online choosing of SV is not feasible, a preselection from all possible SVs was done and 24 sequences of five active switching combinations is used. [[1]]
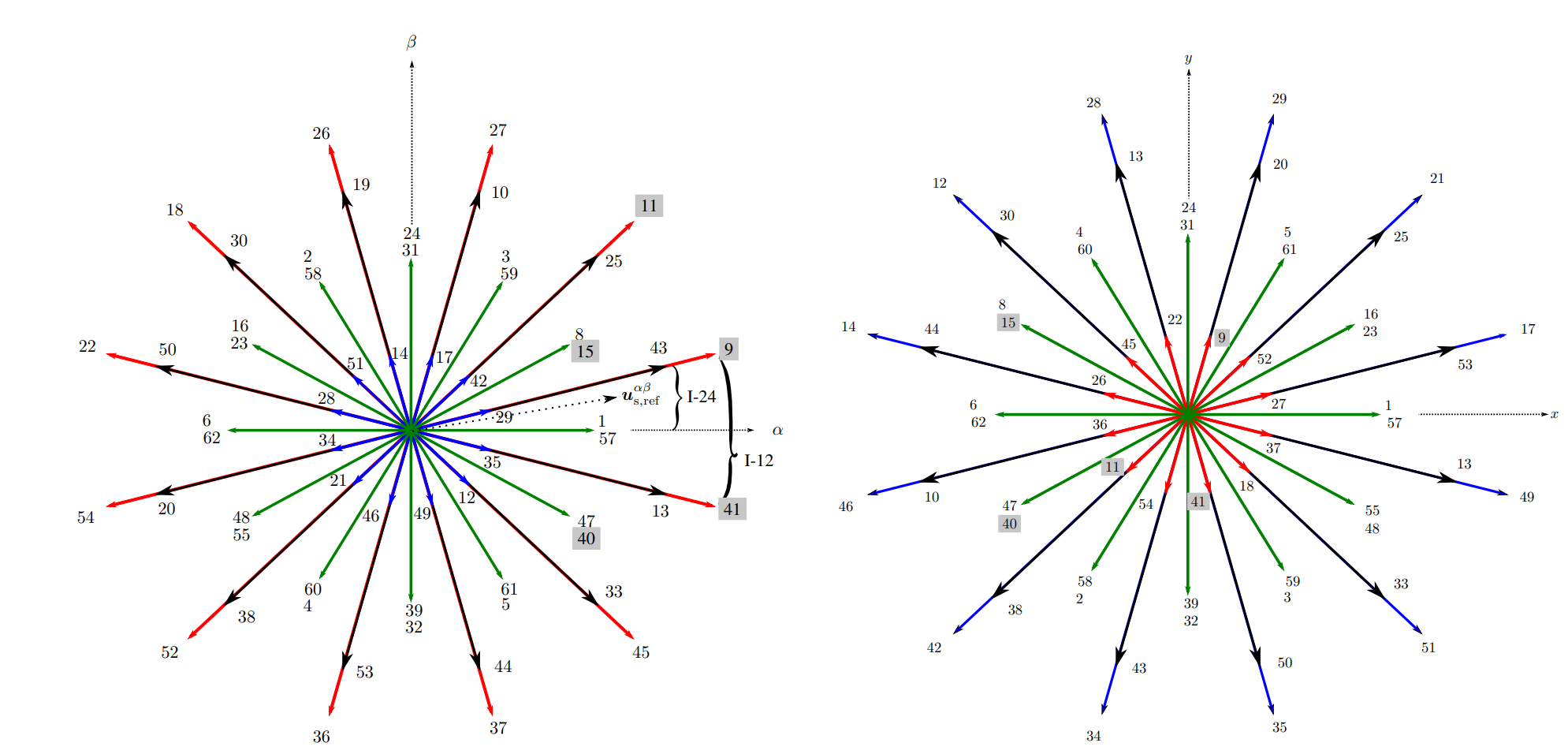
Fig. 308 Selected actives witching combinations in \(\alpha\beta\) (left) and \(XY\) (right) with highlighting for sector 1 (Fig.~2[[2]])#
Usage#
Function references#
-
struct uz_svm_asym_6ph_CSVPWM24_out#
output struct for SVM 6ph
Public Members
-
struct uz_DutyCycle_2x3ph_t Duty_Cycle#
Duty Cycles for PWM module
-
float shift_system1#
phase shift for PWM module system 1 (can be 0 or 0.5)
-
float shift_system2#
phase shift for PWM module system 2 (can be 0 or 0.5)
-
bool limited_alphabeta#
limited flag for u_ref_V in alpha beta
-
bool limited_xy#
limited flag for u_ref_V in xy
-
struct uz_DutyCycle_2x3ph_t Duty_Cycle#
-
struct uz_svm_asym_6ph_CSVPWM24_out uz_Space_Vector_Modulation_asym_6ph_CSVPWM24_alphabeta(uz_6ph_alphabeta_t u_ref_V, float V_dc)#
SVM 6ph with stationary input.
- Parameters:
u_ref_V – reference voltages (controller output)
V_dc – DC voltage (must be positive)
- Returns:
Duty cycles, limited flags and phase shift for PWM modules
-
struct uz_svm_asym_6ph_CSVPWM24_out uz_Space_Vector_Modulation_asym_6ph_CSVPWM24_dq(uz_6ph_dq_t u_ref_V, float V_dc, float theta_el)#
SVM 6ph with rotating input.
- Parameters:
u_ref_V – reference voltages (controller output)
V_dc – DC voltage (must be positive)
theta_el – electric rotor angle
- Returns:
Duty cycles, limited flags and phase shift for PWM modules
Minimum code example#
A minimal code example is given in the following. The SVM not only calculates Duty Cycles, it also yields phase shifts for the PWM IP-Cores. Applying them to the modules is vital. Additionally, the output struct contains two flags, indicating that either the \(\alpha\beta\)- or \(XY\)-setpoints or both have been limited. They can be used for a clamping feature, inhibiting integrators in the control algorithm to overflow. In this example, they are not used.
// declarations
#include "../uz/uz_Space_Vector_Modulation_6ph/uz_Space_Vector_Modulation_6ph.h"
uz_6ph_dq_t v_ref_6ph = {0};
uz_3ph_dq_t v_ref_3ph = {0};
uz_3ph_dq_t cc_setpoint = {0};
struct uz_svm_asym_6ph_CSVPWM24_out svm_out = {0};
...
// in isr
if (current_state==control_state)
{
// example current control
v_ref_3ph = uz_CurrentControl_sample(Global_Data.objects.CC_dq_instance, cc_setpoint, Global_Data.av.actual_3ph_dq, Global_Data.av.v_dc1, Global_Data.av.omega_elec);
v_ref_6ph.d = v_ref_3ph.d;
v_ref_6ph.q = v_ref_3ph.q;
// Modulation
svm_out = uz_Space_Vector_Modulation_asym_6ph_CSVPWM24_dq(v_ref_6ph, Global_Data.av.theta_elec, Global_Data.av.v_dc1);
}
// PWM phase shift
uz_PWM_SS_2L_set_triangle_shift(Global_Data.objects.pwm_d1_pin_0_to_5, svm_out.shift_system1, svm_out.shift_system1, svm_out.shift_system1);
uz_PWM_SS_2L_set_triangle_shift(Global_Data.objects.pwm_d1_pin_6_to_11, svm_out.shift_system2, svm_out.shift_system2, svm_out.shift_system2);
// assign Duty Cycles
uz_PWM_SS_2L_set_duty_cycle(Global_Data.objects.pwm_d1_pin_0_to_5, svm_out.Duty_Cycle.system1.DutyCycle_A, svm_out.Duty_Cycle.system1.DutyCycle_B, svm_out.Duty_Cycle.system1.DutyCycle_C);
uz_PWM_SS_2L_set_duty_cycle(Global_Data.objects.pwm_d1_pin_6_to_11, svm_out.Duty_Cycle.system2.DutyCycle_A, svm_out.Duty_Cycle.system2.DutyCycle_B, svm_out.Duty_Cycle.system2.DutyCycle_C);
Theoretical details#
Limitation#
The 6ph SVM uses two setpoint limitations. Both of them limit the absolute value (length) of the SV, while leaving the phase angle untouched. The checks for the limitations are executed in the listed order below:
The \(XY\)-SV must not be longer than 10% of the \(\alpha\beta\)-SV. As can be seen from (Fig. 3.3[[1]]), a large \(\alpha\beta\)-SV represents a small \(XY\)-SV and vice versa. Therefore, if the \(\alpha\beta\)-SV is near the maximum, the \(XY\)-SV can only be small. Although a general limitation can not achieve maximum usage in all operating points, with this rule an overall good performance can be expected.
Since the maximum voltage is given by the DC-Bus-Voltage and the modulation index \(m_i=\frac{1}{\sqrt{3}}\) (determined in simulation), the combined length of the space vectors has to be limited. Therefore, both lengths are added and if they exceed the maximum allowed voltage, they will be shortened in their existing relations, meaning \(\frac{|SV_{\alpha\beta\textrm{, old}}|}{|SV_\textrm{XY, old}|}=\frac{|SV_{\alpha\beta\textrm{, limited}|}}{|SV_\textrm{XY, limited}|}\) . Since the \(XY\)-SV limitation is executed before and the relation of the \(\alpha\beta\)-SV and \(XY\)-SV are kept the same, the previous limitation will not be violated.
Space vectors#
From the existing \(2^6\) SVs in a six-phase system, not all of them are used. Instead, the selected sequences of (Tab. 3.III[[1]]) are used. Please note that the decimal notation of [[2]] and [[3]] are used. Therefore, the binary values of [[1]] must be mirrored, making the switch a1 being represented by the LSB and c2 by the MSB.
Sector |
Sequence |
---|---|
1 |
[56 - 40 - 41 - 9 - 11 - 15] |
2 |
[56 - 57 - 41 - 9 - 11 - 3] |
3 |
[ 0 - 1 - 9 - 11 - 27 - 59] |
4 |
[ 0 - 8 - 9 - 11 - 27 - 31] |
5 |
[ 7 - 15 - 11 - 27 - 26 - 24] |
6 |
[ 7 - 3 - 11 - 27 - 26 - 58] |
7 |
[63 - 59 - 27 - 26 - 18 - 2] |
8 |
[63 - 31 - 27 - 26 - 18 - 16] |
9 |
[56 - 24 - 26 - 18 - 22 - 23] |
10 |
[56 - 58 - 26 - 18 - 22 - 6] |
11 |
[ 0 - 2 - 18 - 22 - 54 - 62] |
12 |
[ 0 - 16 - 18 - 22 - 54 - 55] |
13 |
[ 7 - 23 - 22 - 54 - 52 - 48] |
14 |
[ 7 - 6 - 22 - 54 - 52 - 60] |
15 |
[63 - 62 - 54 - 52 - 36 - 4] |
16 |
[63 - 55 - 54 - 52 - 36 - 32] |
17 |
[56 - 48 - 52 - 36 - 37 - 39] |
18 |
[56 - 60 - 52 - 36 - 37 - 5] |
19 |
[ 0 - 4 - 36 - 37 - 45 - 61] |
20 |
[ 0 - 32 - 36 - 37 - 45 - 47] |
21 |
[ 7 - 39 - 37 - 45 - 41 - 40] |
22 |
[ 7 - 5 - 37 - 45 - 41 - 57] |
23 |
[63 - 61 - 45 - 41 - 9 - 1] |
24 |
[63 - 47 - 45 - 41 - 9 - 8] |
Offline calculations#
The computation of the Dwell times is done with the voltage references and the inverse matrix of the subsystem components of each SV, as shown in the following equation: [[1]]
Solving the equation for the Dwell times yields: [[1]]
To avoid intensive calculations at runtime, the matrix \([T_\textrm{tv}]^{-1}\) can be precalculated for each sector and stored in the uz_Space_Vector_Modulation_6ph.c
file as static const float inverse_T_tv_all[24][5][5]
.
Later in the calculation, Duty Cycles must be computed with the Dwell times, requiring a specific assignment order for each sector to the respective phase.
In the same way, they are precalculated and stored as static const int svm_offline_order[24][6]
.
The calculations were done in Matlab and all necessary scripts are attached in svm_multiphase_matlab.zip
in the directory of this docs page.
Verification#
Limitation#
To test the limitation, a list of \(\alpha\beta\)-SVs and \(XY\)-SVs was created with \(k \cdot e^{i \cdot \phi}\) and \(k=0, 0.001, ... 1\) und \(\phi=0, 0.001, ... 2\pi\). Each \(\alpha\beta\)-SV was combined with each \(XY\)-SV and applied to the SVM. Using no limitation, the SVM threw an error for a Duty Cycle out of range (negative or greater 1). Using the limitation, no error occured and the relative limit of \(XY\)-SV to \(\alpha\beta\)-SV could even be raised up to 50% without causing an invalid Duty Cycle.
Open loop simulation#
To verify the SVM in open loop simulation, setpoint voltages for \(\alpha\beta\) and \(XY\) given to the SVM. The resulting Duty Cycles were fed into the PWM-IP-Core Simulink model and connected to Simscape VSI-models with \(V_\textrm{DC}=1000\textrm{ V}\) and a resistive six-phase load with 2N.
Setpoints and measured output voltages are shown in the following figure for both subspaces. In the last row, the limit flags for both subspaces are shown.
Fig. 309 Open loop simulation#
Closed loop testbench#
On the testbench, a comparison between SPWM and SVM was conducted. For this test, an asymmetrical six-phase PMSM (\(\Psi_\textrm{PM}=0.0047\textrm{ Vs}\)) was operated with 2N and \(V_\textrm{DC}=3\textrm{ V}\). The machine is externally driven and the speed, starting at \(n_\textrm{mech}=500 \frac{1}{\textrm{min}}\) was increased in increments of \(10\frac{1}{\textrm{min}}\). Current controllers in \(dq\) are set to zero, no other controls are active.
In the resulting figure (see below), a limit flag can be seen. For SPWM this flag is the current controllers external clamping flag. It occurs at \(n_\textrm{mech}=570\frac{1}{\textrm{min}}\) and is accompanied by the currents that can no longer be controlled to zero.
For SVM the limited_alphabeta
flag of the output struct is used.
Since it has a small tolerance (2.5%), it is high at \(n_\textrm{mech}=630\frac{1}{\textrm{min}}\), whereas the currents are still at zero.
Only at \(n_\textrm{mech}=660\frac{1}{\textrm{min}}\) the current controllers cannot keep the currents to zero anymore.
This is also the expected behavior, as the reachable speed with SVM should be \(n_\textrm{mech,SVM}=n_\textrm{mech,SPWM} \cdot\frac{2}{\sqrt{3}}\approx 660\frac{1}{\textrm{min}}\).
Fig. 310 Testbench comparison of SPWM and SVM#